Query (query) is the most commonly used term in WordPress to refer to queries sent to the database to get the information of a Post (or Page, or whatever). If you explain the query in detail, it is very long, but in this article you only understand that Query is a set of SQL commands sent to MySQL Server to get the data of WordPress posts.
In this article, I will guide you to learn about the default queries in WordPress on each page, and how to create a new query to the database through the class. WP_Query.
How to query on each page
By default, every page on WordPress contains a query, for example on the homepage it will send a query to get the list of the latest posts, when entering the article content page, it will send a query to get the information of that article based on in the ID of the article (in the previous section, I said that WordPress can parse a query on a page based on the access path).
But to see exactly how many queries are on your page, and how it sends, we have to debug to see. The easiest way is to install the plugin Debug Bar then insert this paragraph wp-config.php:
define( ‘SAVEQUERIES’, true );
Then you go to the homepage, click the Debug button on the Admin Bar and look at the WP Query section.
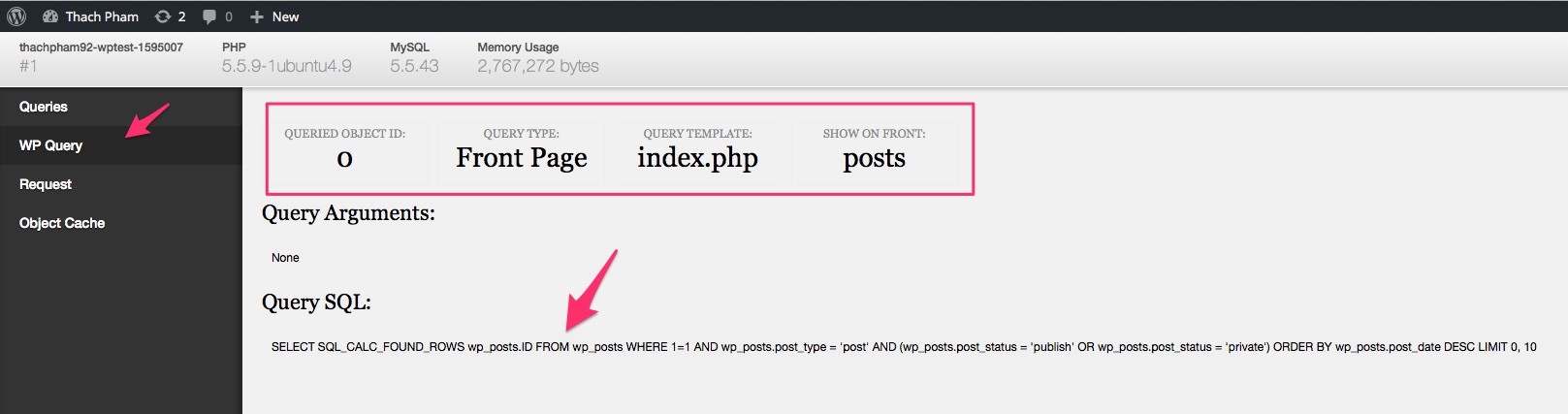
View page query with Debug Bar
In it, the above section is important information about the current page’s query such as the template that is executing the query (the files in the theme), the type of query. The remaining part Query SQL is the SQL command that executes that query. If you’ve ever worked with SQL, you’ll surely understand what that paragraph means. As shown in the figure, it takes the data in the wp_posts table, the order number is 1 and the post_type column is post and post_status is publish and sorts it based on the post_date column value in ascending order with the number of 10 objects. But don’t worry about these things because WordPress supports us to create the fastest query without having to remember the table name, column name at all.
Similarly, you can go to other sites and see their queries.
Where are the results of the query stored?
After submitting the query, there must be a return result of that query. For example, when it sends a query to get a new post, it will certainly return the information of the new post that the query is looking for. And the results of the query will be saved to the object $wp_query
.
Now try to lookup the $wp_query object by inserting the following code at the end of the template footer.php in the theme:
<?php
echo ‘<pre>’;
print_r ($wp_query);
echo ‘</pre>’;
?>
And now, you can go through certain posts or pages to see the audience’s information $wp_query
after it has sent the query, it will include all the information it received (if it’s left blank, it doesn’t).
However, you guys notice the most for yourself in the attribute posts
.
And if now you only need to see the data of the posts attribute then fix the debug code pointing to the property posts
in the object $wp_query.
<?php
echo ‘<pre>’;
print_r ($wp_query->posts);
echo ‘</pre>’;
?>
But the good news for you is the $wp_query->posts
has a private object named $posts
so maybe you just need to lookup the object $posts
is out.
<?php
echo ‘<pre>’;
print_r ($posts);
echo ‘</pre>’;
?>
And as you can see, we can now see the properties in the object $posts
information about the article.
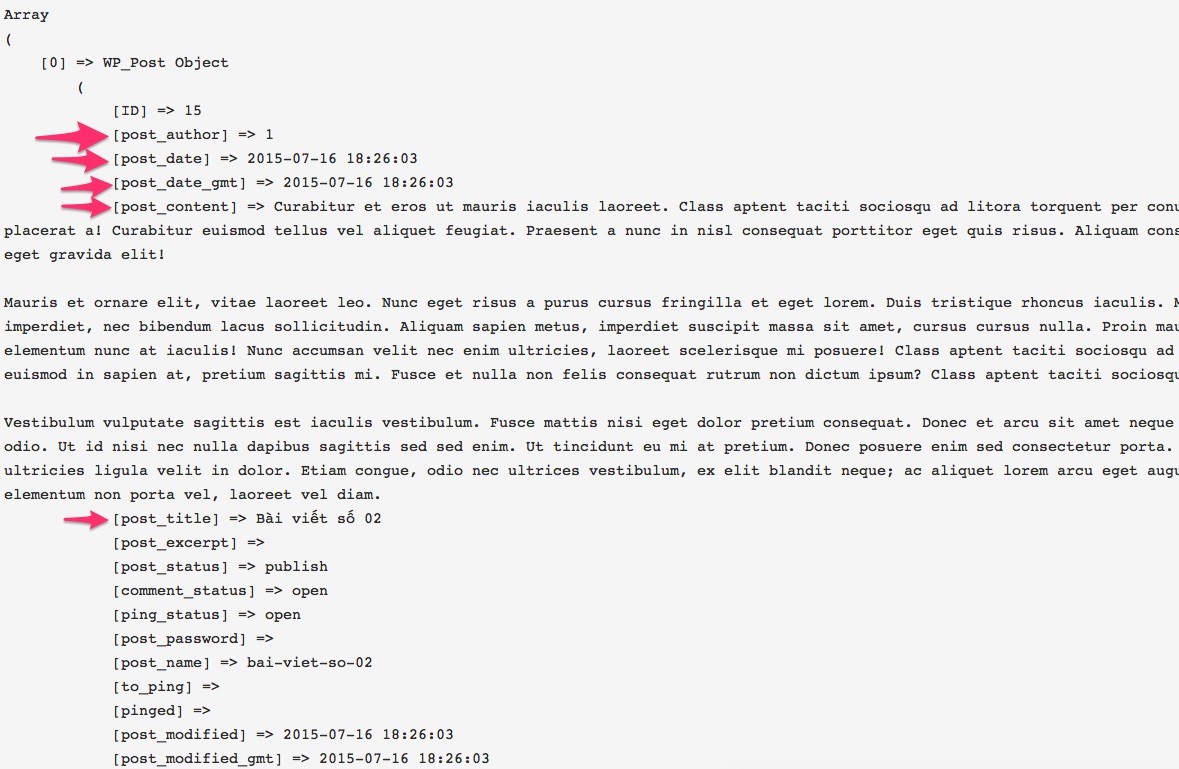
Properties in the $posts . object
However if you need to retrieve, we should get by object $post
(no s at the end). Now try opening the template again footer.phptry lookup the attribute post_content
in the object $post
let’s see. Or you can put it in any template in the theme, in any position.
<?php
echo ‘<pre>’;
print_r ($post->post_content);
echo ‘</pre>’;
?>
Use loop for query
And if you do it right when printing $post->post_content
then it will return the content of the current post if you go to view the content of a post. But if you look outside the index page, or archive pages like tag, category, etc., it only displays the first post content. Why?
Because the $post is actually an object in an array (array), so if you call it like that, PHP will understand that we only want to call the first object in the array, so when viewing pages with many posts, it also shows only one post. And let it show in its entirety, we must create a loop (loop) for that object array so that it retrieves multiple articles. And we will iterate through the method have_posts()
in the object $wp_query
.
Before diving deeper, let’s understand the structure of a loop in WordPress, and its structure is as follows:
<?php
if( $wp_query->have_posts() ) { // Nếu phương thức have_posts() trả về TRUE thì mới chạy code bên trong
while( $wp_query->have_posts() ) { // Nếu have_posts() == TRUE thì nó mới lặp, không thì ngừng
$wp_query->the_post(); // Thiết lập số thứ tự bài viết trong chỉ mục của query/*
* Nội dung hiển thị bài viết
*/
echo $post->post_title . ‘<br>’;}
}
?>
I write that for you to understand, but usually, WordPress often shortens it to like this:
<?php
if( $wp_query->have_posts() ) : while( $wp_query->have_posts() ) : $wp_query->the_post();/*
* Nội dung hiển thị bài viết
*/
echo $post->post_title . ‘<br>’;endwhile; endif;
?>
You put this somewhere in the template like in footer.php for example, then go to the homepage or archive page and see that you will see it call up the post titles of many different articles.
What does it mean, please read the comments and see more details of the methods below.
Meaning of have_posts() method
Method have_posts()
is a method of class WP_Query
which creates the object $wp_query
. This method is declared in the file /wp-includes/query.php at lines 3739 – 3767.
/**
* Whether there are more posts available in the loop.
*
* Calls action ‘loop_end’, when the loop is complete.
*
* @since 1.5.0
* @access public
*
* @return bool True if posts are available, false if end of loop.
*/
public function have_posts() {
if ( $this->current_post + 1 < $this->post_count ) {
return true;
} elseif ( $this->current_post + 1 == $this->post_count && $this->post_count > 0 ) {
/**
* Fires once the loop has ended.
*
* @since 2.0.0
*
* @param WP_Query &$this The WP_Query instance (passed by reference).
*/
do_action_ref_array( ‘loop_end’, array( &$this ) );
// Do some cleaning up after the loop
$this->rewind_posts();
}$this->in_the_loop = false;
return false;
}
The meaning of this method is very simple, that is it will Check to see if there are any posts in the query. The reason it can be checked is that it takes the current article number (attribute ). current_post
) plus 1 unit that is less than the total number of posts in the query (attribute post_count
) then it will return true. The opposite is FALSE.
And this paragraph:
if( $wp_query->have_posts() )
i.e. it checks if it has posts in current query ($wp_query) then it executes below loop.
while( $wp_query->have_posts() )
And in this loop, it will loop if have_posts() returns true. If false then it will stop.
the_post() method
After calling the loop out, you can see it has more methods the_post()
inside. So what is this?
This method is declared in /wp-includes/query.php at lines 3712 – 3737.
/**
* Sets up the current post.
*
* Retrieves the next post, sets up the post, sets the ‘in the loop’
* property to true.
*
* @since 1.5.0
* @access public
*/
public function the_post() {
global $post;
$this->in_the_loop = true;if ( $this->current_post == -1 ) // loop has just started
/**
* Fires once the loop is started.
*
* @since 2.0.0
*
* @param WP_Query &$this The WP_Query instance (passed by reference).
*/
do_action_ref_array( ‘loop_start’, array( &$this ) );$post = $this->next_post();
$this->setup_postdata( $post );
}
This method means that it will index the order of the posts in the loop and the specific function is to set the in_the_loop property to true because the default is false, which if it is true then the next_post() method has executable to get the next article.
So in conclusion, this method will be functional count the index to call the next post in the loopif you don’t declare this method in the loop then the first post will be repeated over and over again without stopping.
$wp_query does not need to be declared in the loop
$wp_query is the object that contains the default query on the page, so you may not need to call it in the loop, it will understand that you are looping the default query.
<?php
if( have_posts() ) : while( have_posts() ) : the_post();/*
* Nội dung hiển thị bài viết
*/
echo $post->post_title . ‘<br>’;endwhile; endif;
?>
Using template tags in a loop
In the loop, if you need to get the post information, you can not use the $post object, but just declare the functions template tag (see previous post) only. It will understand you get the object in the query it is iterating (see file content /wp-includes/post-template.php For more details).
<?php
if( have_posts() ) : while( have_posts() ) : the_post();/*
* Nội dung hiển thị bài viết
*/
the_title();endwhile; endif;
?>
Example of a complete loop
By combining with HTML, we can create a loop to get the list of posts like this:
<?php
if( have_posts() ) : while ( have_posts() ) : the_post();
?> <!–Đóng PHP để viết HTML tiện hơn ở dưới–><!–Nội dung một bài viết–>
<article <?php post_class() ?> >
<h1><a href="https://TechtipsNReview.com/wordpress/wordpress-development/<?php the_permalink(); ?>"><?php the_title(); ?></a></h1>
<summary>
<?php the_content(); ?>
</summary>
<footer>
<?php the_tags(); ?>
</footer>
</article>
<!–kết thúc nội dung–><?php endwhile; endif; // Kết thúc vòng lặp ?>
I’ll say it right here, tags like <article>
,<summary>
,<footer>
same card <div>
well, those are HTML5 tags so they look more structured.
Create a new query object with WP_Query
After looking at the above, you know WordPress has a default query per page stored in the $wp_query object. But fortunately, you can create many other query objects with custom parameters that get the posts as you want, that is we will use the WP_Query class.
Using
How to use this class is quite simple, that is to create it into a variable as an object and declare the parameters (array form) to customize the article.
$my_query = new WP_Query( $args );
In there, $args
is the variable that holds the parameter. For example, I can declare parameters and create a query like this:
<?php
$args_my_query = array(
‘post_type’ => ‘post’,
‘orderby’ => ‘rand’
);
$my_query = new WP_Query( $args_my_query );
?>
And then you bring the object $my_query
just put it in the loop.
<?php
if( $my_query->have_posts() ) : while( $my_query->have_posts() ) : $my_query->the_post();/*
* Nội dung hiển thị bài viết
*/
the_title();endwhile; endif;
?>
Here I will not talk about creating a new query with pagination, but I will talk about this in a separate article.
List of parameters of WP_Query
The power of WP_Query is in the parameters. With a diverse and rich number of parameters, you can get any type of article anywhere on the website. The parameters I have gathered into a note, you can refer to https://gist.github.com/thachpham92/d57b18cf02e3550acdb5.
Epilogue
This article may be a bit long, but that is the most important thing about WP Query that I want to tell you. By mastering how to use WP Query and understanding queries in WordPress, you can do a lot of things because almost in WordPress we use WP Query a lot.
Source: Use WP Query and Loop to get posts
– TechtipsnReview