AJAX (Asynchronous JavaScript and XML) technique is now too popular on the website, it will help to transmit data from the backend to the frontend without reloading the page. Every day you will probably see many examples of AJAX usage, like clicking the like button or sending a comment on Facebook are also AJAX.
In this article, I will show you how to familiarize yourself with AJAX techniques applied to WordPress with the most common example being AJAX pagination. From this example, you can understand how AJAX works in WordPress to send data to the front-end.
Download source
Use the TwentyFifteen theme
In this example, I will use the TwentyFifteen theme so that it is easy for you to follow. But you can still do it in other themes because you just need to find the selection of the redirect link only, if you customize it from another theme, you should create a child theme.
Create plugin folder
Now go to the directory /wp-content/plugins/ and create another folder named ajax-pagination. In the folder ajax-paginationyou create a file named plugin.php with the following content:
<?php
/*
Plugin Name: AJAX Pagination
Description: Phân trang website bằng AJAX
Version: 1.0
Author: Thach Pham
Author URI: https://TechtipsNReview.com
*/
From now on, all your PHP code will be written in this plugin.php file. Then you can go to Plugins -> Installed Plugins and activate this plugin.
Embed Javascript file in theme
When working with AJAX we will need to write some more Javascript, so we will need to create a .js file in the plugin and then write the code for it to embed itself in the theme. Now create a file named ajax-pagination.jsthen write this code to the plugin.php file to insert this file into the theme you are using automatically through the function wp_enqueue_script
then hook it on the hook wp_enqueue_scripts
let it execute.
/*
@ ajax_pagination_scripts()
@ Nhúng file ajax-pagination.js vào theme
*/
add_action( ‘wp_enqueue_scripts’, ‘ajax_pagination_scripts’ );
function ajax_pagination_scripts() {/*
* Chèn file ajax-pagination.js vào frontend
*/
wp_enqueue_script( ‘ajax-pagination-script’, plugins_url( ‘/ajax-pagination.js’, __FILE__ ),
array( ‘jquery’ )
);
}
About how hooks work wp_enqueue_scripts
and function wp_enqueue_script
I will explain how in another post. But first of all now you can understand that with the above paragraph it will automatically insert a file ajax-pagination.js in its current plugin directory thanks to the function plugins_url()
female array('jquery')
which means for WordPress to understand this script will use jQuery to automatically insert into the theme and always load after jQuery to make it error.
If you need to insert many different Javascript files, just write multiple paragraphs wp_enqueue_script()
in a function and hook it into a hook wp_enqueue_scripts
is to be.
Now go to the website to check the HTML source code outside its frontend, the file ajax-pagination.js has been called like this.
Find the pagination class and write events for AJAX
Event for AJAX means an action that we want to rely on to trigger the submission of the query, here we will use the click event to send the query when a certain location is clicked. And that position we will define is the link inside the pagination bar.
Now go to the paging bar, right-click on the link and select Inspect Element (or Firebug) to see a selection of that area, it looks like this.
Notice as shown in the picture, the pagination bar will be inside a selection with class .nav-links
. Then the internal links will have the class of .page-numbers
. Particularly for the link for the next button, there will be more classes .next
. So to generate the correct event, we will set up a click event based on the a tag in the selection .nav-links
(written as .nav-links a
).
First write this to the file ajax-pagination.js to set the event and test it.
// chắc chắn là đang sử dụng chế độ No Conflict của jQuery, sử dụng jQuery() thay vì $()
jQuery.noConflict();// load sau khi website được tải xong
jQuery( document ).ready( function($) {$(document).on ( ‘click’, ‘.nav-links a’, function( event ) {
event.preventDefault();
// kiểm tra event click
alert( "Bạn vừa click vào phân trang đấy!" );
} ) // end event} );
And now try to click on the pagination to see if you can see the alert, if you see it, then it is successful and delete the paragraph alert()
in the code.
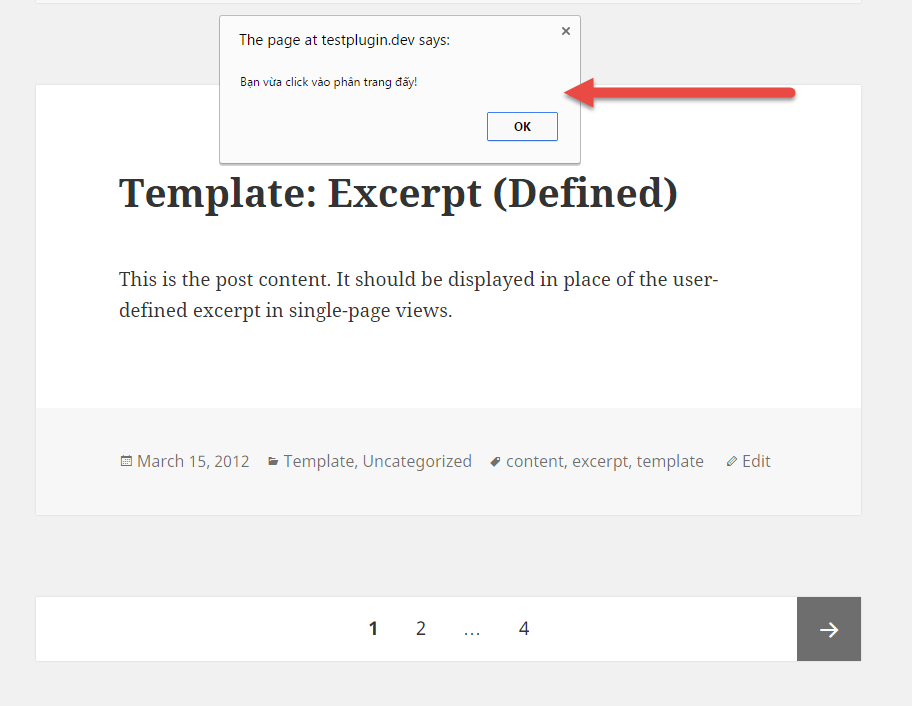
Event testing
Using AJAX in WordPress
By default, WordPress has built-in methods to process data with AJAX, so we only need to call it if we use it. To invoke it, we will proceed to call file admin-ajax.php available in WordPress with the following code (placed inside the function ajax_pagination_scripts
which we declared above):
/*
* Gọi AJAX trong WordPress
*/
global $wp_query;
wp_localize_script( ‘ajax-pagination-script’, ‘ajax_object’, array(// Các phương thức sẽ sử dụng
‘ajax_url’ => admin_url( ‘admin-ajax.php’ ),
‘query_vars’ => json_encode( $wp_query->query )));
Jaw wp_localize_script()
will have the effect of detecting and using a certain script already in the WordPress source code because the file admin-ajax.php already available so don’t use wp_enqueue_script()
as above. In there, ajax_object
is the object that we will use later in the .js file to define the AJAX object. Still global $wp_query;
is that we will give the object $wp_query
global so that it can be used in the plugin’s file to get the post’s data.
After inserting, you check the source and you will see a script printed as follows:
[html]
[/html]
Now the rest that you need to do in this step is to write Javascript to declare AJAX.
// chắc chắn là đang sử dụng chế độ No Conflict của jQuery, sử dụng jQuery() thay vì $()
jQuery.noConflict();// load sau khi website được tải xong
jQuery( document ).ready( function($) {$(document).on ( ‘click’, ‘.nav-links a’, function( event ) {
event.preventDefault();// AJAX
$.ajax({
url: ajax_object.ajax_url,
type: ‘post’,
data: { action: ‘ajax_pagination_data’ },
success: function( ketqua ) {// Thử nghiệm
var ketqua = "AJAX working";
alert( ketqua );}
})
} ) // end event} );
From paragraphs 10 to 23 are the codes that we need to add to the ajax-pagination.js file, I explain as follows:
$.ajax()
– This is a special function in jQuery to make AJAX handling simpler and cleaner.url
: The path of the file to which the data will be sent when executing AJAX from the click event. ajax_object.ajax_url means the path of the admin-ajax.php file that we declared in the plugin.php file.type
: the type of method to send data, use POST or GET whatever you want, but if it’s just receiving data, I recommend you to use POST.data
: declares the object containing the data returned after sending the request from AJAX. ajax_pagination_data means the function that returns data, we will write it later.success
: action after AJAX data transmission is successful, temporarily here will test by printing a piece of text for testing. If it returns, then success.
Now save it and try to click on the pagination link to see if the ketqua variable returns, if it does, then it works.
Create a function that returns data from WordPress
As I said above, the data parameter will contain the return object, so now we will build the ajax_pagination_data object to return to the user when they transmit data. First you will need to edit the file ajax-pagination.js become as follows:
// chắc chắn là đang sử dụng chế độ No Conflict của jQuery, sử dụng jQuery() thay vì $()
jQuery.noConflict();// load sau khi website được tải xong
jQuery( document ).ready( function($) {/*
@ hàm xác định số trang sẽ được tải
@ bằng cách bóc tách số trong chuỗi dữ liệu
*/
function set_page( element ) {
element.find(‘span’).remove();
return parseInt( element.html() );
}$(document).on ( ‘click’, ‘.nav-links a’, function( event ) {
event.preventDefault();
page = set_page( $(this).clone() );// AJAX
$.ajax({
url: ajax_object.ajax_url,
type: ‘post’,
data: {
action: ‘ajax_pagination_data’,
query_vars: ajax_object.query_vars,
page: page
},
beforeSend: function() {
/*
@ Tạo các hiệu ứng trước khi request gửi đi
*/
$( ‘#main’ ).find( ‘article’ ).remove();
$( ‘#main nav’ ).remove();
$( ‘#main’ ).scrollTop(0);
$( ‘#main’ ).append( ‘<div id="loading">Đang lấy dữ liệu bài viết</div>’ );
},
success: function( ketqua ) {
/*
@ Xóa nút loading
@ và khôi phục lại dữ liệu trả về
*/
$( ‘#main’ ).find( ‘#loading’ ).remove();
$( ‘#main’ ).append( ketqua );
console.log(page);}
})
} ) // end event} );
In the new code, we have a new function named set_page()
in Javascript. This function will have the effect of finding all content in the document’s span tag. Then proceed to use the function parseInt()
in Javascript to split the number in the found data string. Its purpose is for us to get the number of the page in the text on the website, for example on the pagination bar there will be the numbers 1,2,3,4,…it will take these numbers to be the value of the page. The page parameter is to help the WordPress query understand which page to get data from.
We will declare more parameters query_vars
and page
which will be used in submitting the query in WordPress to get the list of posts. Parameters query_vars
treated as a method of the object ajax_object
which we have declared in the plugin.php file. The page parameter is that it will use the return value of the set_page() function we created above.
Next is the parameter beforeSend
to add something before the query’s data is sent. We will use it to delete posts ( aritlce
in #main
of TwentyFifteen), remove the existing pagination frame tag (the nav
have class pagination
in #main
of TwentyFifteen) and finally add the received data inside #main
. And importantly, automatically jump to the top of the website with the scrollTop
and print a piece of text that tells the user that data is being loaded.
Finally, the success parameter will return the result after sending the AJAX query to WordPress because the ketqua parameter WordPress understands itself as the function that collects the returned data. Also delete the word Retrieving the other post data.
I would also like to note the following:
#main
– selection of the frame displaying the list of posts.article
– individual selection of post, you can also use .post class.nav.pagination
– selection for pagination frames.
And next is to create a function to return data to AJAX with the hook as the action name wp_ajax_ajax_pagination_data
where ajax_pagination is the action name that we have declared in ajax-pagination.js, and wp_ajax is a required prefix for the hook when working with AJAX in WordPress.
/*
@ Hàm chứa dữ liệu trả về
*/
add_action( ‘wp_ajax_nopriv_ajax_pagination_data’, ‘set_ajax_pagination_data’ );
add_action( ‘wp_ajax_ajax_pagination_data’, ‘set_ajax_pagination_data’ );
function set_ajax_pagination_data() {$query_vars = json_decode( stripslashes( $_POST[‘query_vars’] ), true );
$query_vars[‘paged’] = $_POST[‘page’];
$posts = new WP_Query( $query_vars );
$GLOBALS[‘wp_query’] = $posts;if( ! $posts->have_posts() ) {
get_template_part( ‘content’, ‘none’ );
}
else {
while ( $posts->have_posts() ) {
$posts->the_post();
get_template_part( ‘content’, get_post_format() );
}
}the_posts_pagination( array(
‘mid_size’ => 5,
‘prev_text’ => __( ‘Previous page’, ‘twentyfifteen’ ),
‘next_text’ => __( ‘Next page’, ‘twentyfifteen’ ),
‘before_page_number’ => ‘<span class="meta-nav screen-reader-text">’ . __( ‘Page’, ‘twentyfifteen’ ) . ‘ </span>’,
) );die();
}
In the above paragraph, we first pass the data in the variable $_POST['query_vars']
returned by AJAX because the function defaults $.ajax()
of jQuery will return the data as JSON and store it in a variable $query_vars
.
Next, we give the value of $_POST['page']
when sent back by AJAX into the variable $query_vars['paged']
to set up pagination for the query.
Finally we create an object $posts
turn away $query_vars
above and call template content.php out to display the content. In the TwentyFifteen theme, this file will contain the code that displays each element of a post and the loop repeats itself. Also insert a new pagination bar with the function the_posts_pagination()
and terminate the process until it has finished looping.
Now you can save it and go out and try clicking on the redirect link in the numbers in the pagination to see if it works or not, if you did it from the beginning up to now it should work.
It should also be noted that from WordPress 4.1 onwards, the function the_posts_pagination()
will display the numeric pagination bar.
Problems encountered in this tutorial
Actually this tutorial I am having a problem that if you click on the numeric link on the pagination it will work, but if you click on the Next button or something like that it returns the home page. Because according to this tutorial, the WordPress page-by-page data will be retrieved by the variable $query_vars['paged']
which it gets from the data that jQuery sends back by AJAX, while in the Javascript file I have specified that the page number will be found using the function parseInt()
in Javascript to separate the number on the tag, which is the link of each page in the pagination. But if the tag has the text Next, of course it will return the value “NaH”, which corresponds to the fact that there is no numeric data, so it will not work.
As for how to do Next, Prev pagination, now there are many tutorials so you can search on Google.
Good luck!
Source: Self-code the pagination function with AJAX
– TechtipsnReview