As a programmer or developer, the importance of creating secure applications cannot be underestimated.
Software security addresses the management of malicious attacks by identifying potential vulnerabilities in software and taking the necessary precautions to protect them.
Software can never be 100% secure because a developer can ignore a bug, create new bugs while fixing existing cases, or create new vulnerabilities through updates.
However, there are two main methods that all software developers can use to ensure that they create secure software. It’s about writing secure code from scratch and testing it effectively.
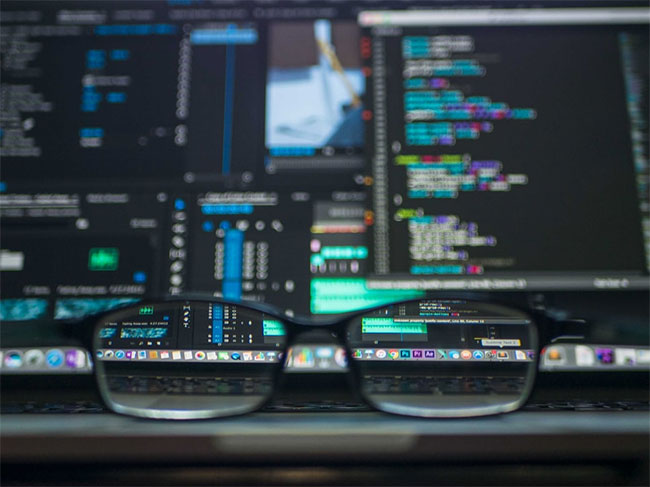
How to write security code
Writing secure code has only one thing to do – it’s error handling. If you can anticipate every potential value a user might give the application and generate a response in the program to that value, then you are writing secure code.
This is much simpler than you might think, as all good developers know almost everything about the applications they develop. Therefore, you should know any values that your application requires to perform a task (approved values) and understand that any other possible values are unapproved values. .
Write security code
Suppose you want to create a program that accepts only two integer values from the user and performs an addition operation on them. As a good developer, you now know everything about your app. You know all the values this program will accept (integer values) and you know the task this program will complete (an addition operation).
Example of creating a program in Java
import java.util.Scanner;
public class Main {
//The main function that executes the program and collects the two values
public static void main(String[] args) {
System.out.println("Please enter your two integer values: ");
int value1;
int value2;
Scanner input = new Scanner(System.in);
value1 = input.nextInt();
value2 = input.nextInt();
addition(value1, value2);
input.close();
}
//the function that collects the two values and displays their sum
private static void addition(int value1, int value2) {
int sum;
sum = value1 + value2;
System.out.println("The sum of the two integer values you entered: "+ sum);
}
}
The above code generates an application that matches the requirements exactly. When executed, it will produce the following line in the console:
Please enter your two integer values:
The application will then remain paused until the user enters two integer values into the console (that is, enter the first value, press the Enter and repeat).
If the user enters the values 5 and 4 in the console, the program will produce the following output:
The sum of the two integer values you entered: 9
That’s great. The program does exactly what it is supposed to do. However, if a nefarious user comes in and enters a non-integer value, such as “g”, into your application, there will be problems. This is because there is no code in the application that protects against unapproved values.
At this point, your application will crash, creating a potential gateway into your application for hackers to know exactly what to do next.
Example of program security
import java.util.InputMismatchException;
import java.util.Scanner;
public class Main {
//The main function that executes the program and collects the two values
public static void main(String[] args) {
try {
System.out.println("Please enter your two integer values: ");
int value1;
int value2;
//using the scanner class to read each input from the user,
//and assign it to is respective variable (throws an exception if the values are not integers)
Scanner input = new Scanner(System.in);
value1 = input.nextInt();
value2 = input.nextInt();
//calls the addition function and passes the two values to it
addition(value1, value2);
//closes the input stream after it has come to the end of its use
input.close();
//handle all the errors thrown in the try block
}catch(InputMismatchException e){
System.out.println("Please enter a valid integer value.");
}catch(Exception e) {
System.out.println(e.getMessage());
}
}
//the function that collects the two values and displays their sum
private static void addition(int value1, int value2) {
int sum;
sum = value1 + value2;
System.out.println("The sum of the two integer values you entered: "+ sum);
}
}
The above code is secure because it implements exception handling. Therefore, if you enter a non-integer value, the program will generate the following line of code:
Please enter a valid integer value.
What is Exception handling?
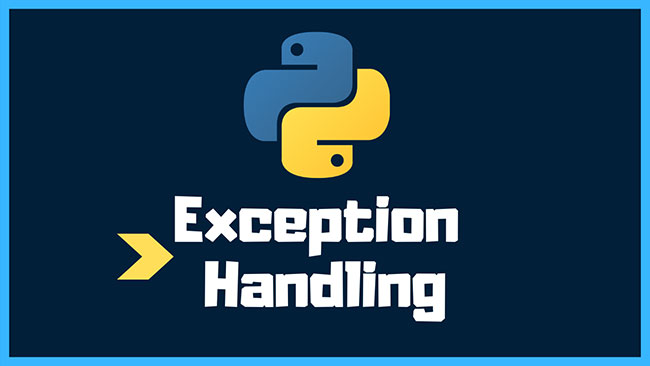
Basically, exception handling is a modern version of error handling where you separate the error handling code from the normal handling code. In the above example, all code that handles normally (or code capable of generating exceptions) is in block try and all error handling code is in block catch.
If you take a closer look at the example above, you’ll see that there are 2 catch blocks. First take an argument InputMismatchException – this is the name of the exception thrown if a non-integer value is entered. Second take the argument Exception and this is important because its purpose is to find any exception in the code that the developer did not find during testing.
Check the code
You should never underestimate the power of testing and re-checking code. Many developers (and application users) find new bugs after the software is made available to the public.
Thorough testing of the code ensures that you know what your application will do in every conceivable situation, and that allows you to protect it from data breaches.
Consider the example above. What if, upon completion, you only test the application with integer values? You may leave the application thinking that you have successfully identified all potential errors when in fact this is not the case.
The reality is that you may not be able to identify all potential errors. This is why error handling works in tandem with testing your code. Testing the program above reveals a potential error that would occur in a particular situation.
However, if some other error that doesn’t appear during the test exists, the second catch block in the code above will handle it.
Database security
If your application connects to a database, the best way to prevent access to that database is to ensure that all aspects of your application are secure. However, what if your application was designed with the sole purpose of providing an interface to said database?
This is where things get a little more interesting. In its most basic form, a database allows users to add, retrieve, update, and delete data. A DBMS is an application that allows users to interact directly with a database.
Most databases contain sensitive data, so to maintain integrity and limit access to this data, one requirement is required – access control.
Access control
Access control seeks to maintain the integrity of the database by defining the types of people who can access the database and restricting the type of access they have. Therefore, a good database management system must be able to record who accessed the database, when, and what they did.
It can also prevent registered users from accessing or editing data with which they are not authorized to interact.
Source link: Why is software security a skill all programmers should have?
– https://techtipsnreview.com/